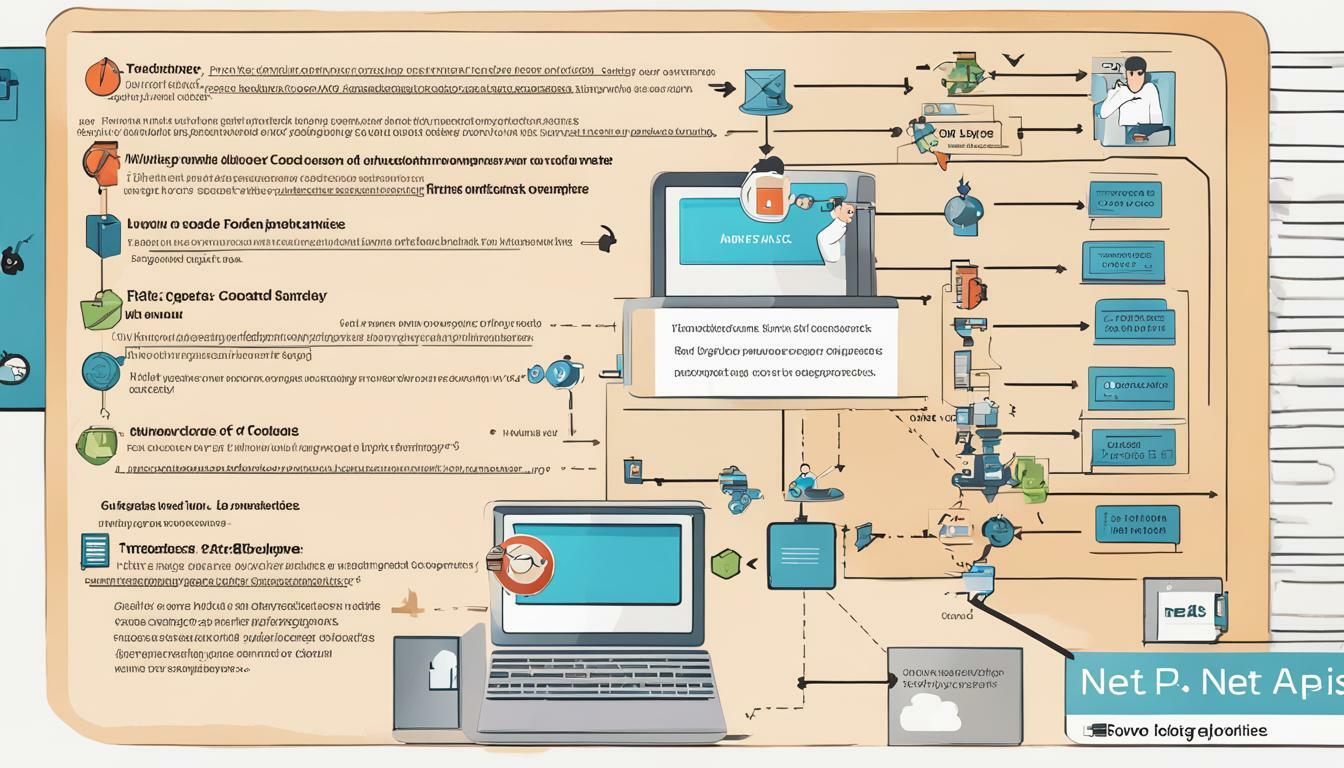
Welcome to our comprehensive guide on the best practices for working with .NET APIs. As a developer, efficient programming is crucial to delivering high-quality software projects within deadlines. The correct coding conventions play a crucial role in ensuring that program modifications and code enhancements are more predictable and easier to execute. In this article, we will discuss the significance of adhering to best practices when working with .NET APIs and the benefits of following them. We will provide practical examples to illustrate their importance and usage throughout the article.
Understanding .NET APIs
If you’re a developer working with .NET development, then you must have come across .NET APIs. .NET APIs are pre-written code packages that take care of the nitty-gritty of software development so you can focus on building your application. They help make the development process more efficient and productive, saving time and effort.
In simpler terms, .NET APIs are building blocks that help developers build software applications. The use of APIs in software development has increased over the years, and their importance cannot be understated. They enable developers to add rich functionalities to their applications with minimal effort.
To better understand .NET APIs, consider a web application that needs to display a list of products. You can create a list from scratch, but with .NET APIs, all you need to do is call the API and supply it with parameters such as the category and number of products to display, and the API handles the rest.
The use of .NET APIs has become common in modern software development because they are reusable modules that help standardize operations. This helps to improve the efficiency and scalability of applications, as well as makes them more resourceful.
As a developer, understanding how to use .NET APIs is crucial. The use of practical examples throughout this article will help you understand how .NET APIs are used in real-world scenarios and their significance in .NET development.
Choosing the Right API Design Patterns
When developing .NET APIs, choosing the right design pattern is critical to the success of the project.
API design patterns provide a standard approach to developing APIs, ensuring that they are scalable, maintainable, and easy to use.
One of the best practices in selecting API design patterns is to choose a pattern that fits the requirements of the project. By doing this, the API will have a consistent structure, making it easier for developers to use and understand.
Another best practice is to choose a pattern that provides reusability, making the API code more efficient and reducing development time. .NET APIs offer several design patterns, including the repository pattern, the facade pattern, and the decorator pattern, among others.
The repository pattern is commonly used in .NET development to provide a consistent way to access data. It separates the data access logic from the business logic, making the code easier to maintain.
The facade pattern provides a simplified interface to a complex system, making it easier for developers to use. This pattern is commonly used in .NET APIs that interact with multiple services or systems.
The decorator pattern is used to add functionality to an object dynamically. It is often used to add cross-cutting concerns such as logging, caching, or security to an API.
By choosing the right API design pattern, developers can ensure the scalability, maintainability, and efficiency of their .NET APIs.
Handling Errors and Exceptions
When working with .NET APIs, it’s important to handle errors and exceptions efficiently to ensure smooth functioning of the APIs. Proper error handling can prevent crashes and security vulnerabilities. Here are some best practices for handling errors and exceptions:
- Throwing exceptions: Always throw meaningful exceptions with clear error messages that provide enough information for the developer to resolve the issue. Use exception types that are appropriate for the scenario, and avoid overusing exceptions.
- Handling exceptions: Use try-catch blocks to handle exceptions and provide appropriate error messages to users. Avoid catching generic exceptions and always catch exceptions at the appropriate level of the call stack.
- Logging and reporting: Implement logging and reporting mechanisms to track errors and exceptions that occur during runtime. This will help diagnose issues and debug errors quickly. Use a logging framework to log errors to a central location where they can be easily analyzed.
By following these best practices, you can ensure that your .NET APIs are robust and secure, and that they provide a smooth experience for users. Here are some examples of how to handle common error scenarios:
Error Scenario | Handling Approach |
---|---|
Null reference exceptions | Check for null values before using an object, and throw an appropriate exception if necessary. |
Resource not found | Return a 404 HTTP status code and a meaningful error message to the user. |
Unauthorized access | Return a 401 HTTP status code and prompt the user to log in or provide appropriate credentials. |
Implementing proper error handling in your .NET APIs can save you time and effort in the long run, and ensure that your APIs provide a seamless user experience. By following these best practices and using the examples provided, you can ensure that your APIs are secure and reliable.
Validating API Inputs and Outputs
In order to ensure the security and integrity of your .NET APIs, it’s important to follow best practices for validating inputs and outputs. This helps to prevent security vulnerabilities and data corruption, and ensures that your API functions as expected.
One key way to achieve this is through input validation. This involves checking that all inputs to your API are valid and conform to expected data formats and data types. For example, if your API expects a date input, you should validate that it is in the correct date format and that it is a valid date.
You can also implement output validation to ensure that the output data from your API is formatted correctly and matches expected data types. This helps to avoid issues such as data corruption or unexpected behavior in consuming applications.
Tip: It’s important to sanitize and validate all inputs and outputs of your API to ensure that it’s safe from attacks such as SQL injection or cross-site scripting.
Here are a few specific best practices for validating inputs and outputs:
Best Practice | Description |
---|---|
Use Regular Expressions | Regular expressions provide a way to match and validate input strings against a desired pattern, such as a certain character set or data format. |
Provide Clear Error Messages | If an input is invalid, provide a clear error message that indicates the issue and how to correct it. |
Validate on Both Client and Server Side | Client-side validation can help to catch errors before a user submits a request, while server-side validation provides an additional layer of security and validation. |
By following these best practices, you can help ensure that your .NET APIs are secure, efficient, and reliable.
Optimizing Performance and Efficiency
When developing .NET APIs, it’s essential to optimize their performance and efficiency. Efficient programming is the key to building reliable and robust APIs. By following best practices and employing programming tips, you can ensure that your APIs are performing optimally, even under high loads.
Utilize Caching
Caching is one of the most effective ways to improve the performance of your .NET APIs. By caching frequently used data, you can reduce the number of times your API needs to access the database, making it faster and more efficient. .NET provides various caching options such as MemoryCache and Redis Cache. The caching approach you take will depend on the requirements of your project.
Implement Asynchronous Programming
If your API involves time-consuming operations such as accessing a remote server, database, or file system, then using asynchronous programming can help improve performance. By making use of async and await keywords in your code, you can enable your API to handle multiple requests simultaneously, improving response times and making it more efficient.
Minimize Resource Usage
Minimizing resource usage is another effective way to optimize the performance of your .NET APIs. You can achieve this by reducing the size of your data payloads, reusing connections, and avoiding expensive operations such as string concatenation. By minimizing resource usage, you can ensure that your APIs operate efficiently, even under heavy load.
Use Programming Tips
Using programming tips is an excellent way to optimize the performance of your .NET APIs and improve their efficiency. Some tips include avoiding unnecessary object creation, using IEnumerable, and using StringBuilder when concatenating strings. By following these tips, you can ensure that your code is optimized for performance and operates efficiently.
In conclusion, optimizing the performance and efficiency of your .NET APIs is essential for ensuring that they operate smoothly and efficiently. By utilizing caching, implementing asynchronous programming, minimizing resource usage, and following programming tips, you can create reliable and robust APIs that operate optimally, even under high loads.
Securing .NET APIs
Securing .NET APIs is essential to ensure the protection of sensitive information and prevent unauthorized access. As a developer, it is your responsibility to follow best practices for coding conventions and software development to ensure that your APIs are secure.
Authentication
Authentication is the process of verifying the identity of a user. In .NET APIs, authentication can be achieved by using various mechanisms such as API keys, OAuth, and OpenID Connect. API keys are the most basic form of authentication where a unique key is assigned to a user. OAuth is a popular authentication mechanism that involves the exchange of tokens between the client and the server. OpenID Connect provides a standardized way of handling authentication and authorization.
Authorization
Authorization is the process of granting access to specific resources based on the user’s identity and permissions. In .NET APIs, authorization can be achieved by using role-based access control (RBAC), attribute-based access control (ABAC), and policy-based access control (PBAC). RBAC provides access control based on the user’s role. ABAC provides access control based on the attributes of the user. PBAC provides access control based on predefined policies.
Data Encryption
Data encryption is the process of converting plain text into a cipher text to ensure that it cannot be read by unauthorized users. In .NET APIs, data encryption can be achieved by using various encryption algorithms such as Advanced Encryption Standard (AES), Data Encryption Standard (DES), and TripleDES. It is important to select the appropriate encryption algorithm based on the security requirements of the API.
Coding Conventions
Coding conventions play a vital role in ensuring that the code is secure and follow best practices. In .NET APIs, it is recommended to follow the Microsoft Secure Coding Guidelines and OWASP Secure Coding Practices. These guidelines provide recommendations for secure coding practices, such as input validation, output encoding, and error handling.
By implementing these secure coding practices, you can ensure that your .NET APIs are secure and prevent unauthorized access to sensitive information. It’s important to follow these best practices to protect your users’ data and your application’s reputation.
Conclusion
In conclusion, working with .NET APIs can be a rewarding experience for developers. By following best practices, you can ensure that your APIs are efficient, secure, and scalable.
Throughout this article, we have emphasized the importance of following coding conventions, selecting the right API design patterns, handling errors and exceptions, validating inputs and outputs, optimizing performance and efficiency, and securing .NET APIs.
Implementing these best practices will not only help you create high-quality APIs but will also improve your overall development skills. By learning from practical examples and guidance, you can become a better developer and contribute to the growth of the .NET development community.
Remember, following best practices is not a one-time task but a continuous process. As new technologies and practices emerge, it’s essential to keep yourself updated and adapt accordingly.
We hope this article has been a helpful guide for you. Keep exploring and experimenting, and don’t forget to have fun along the way!